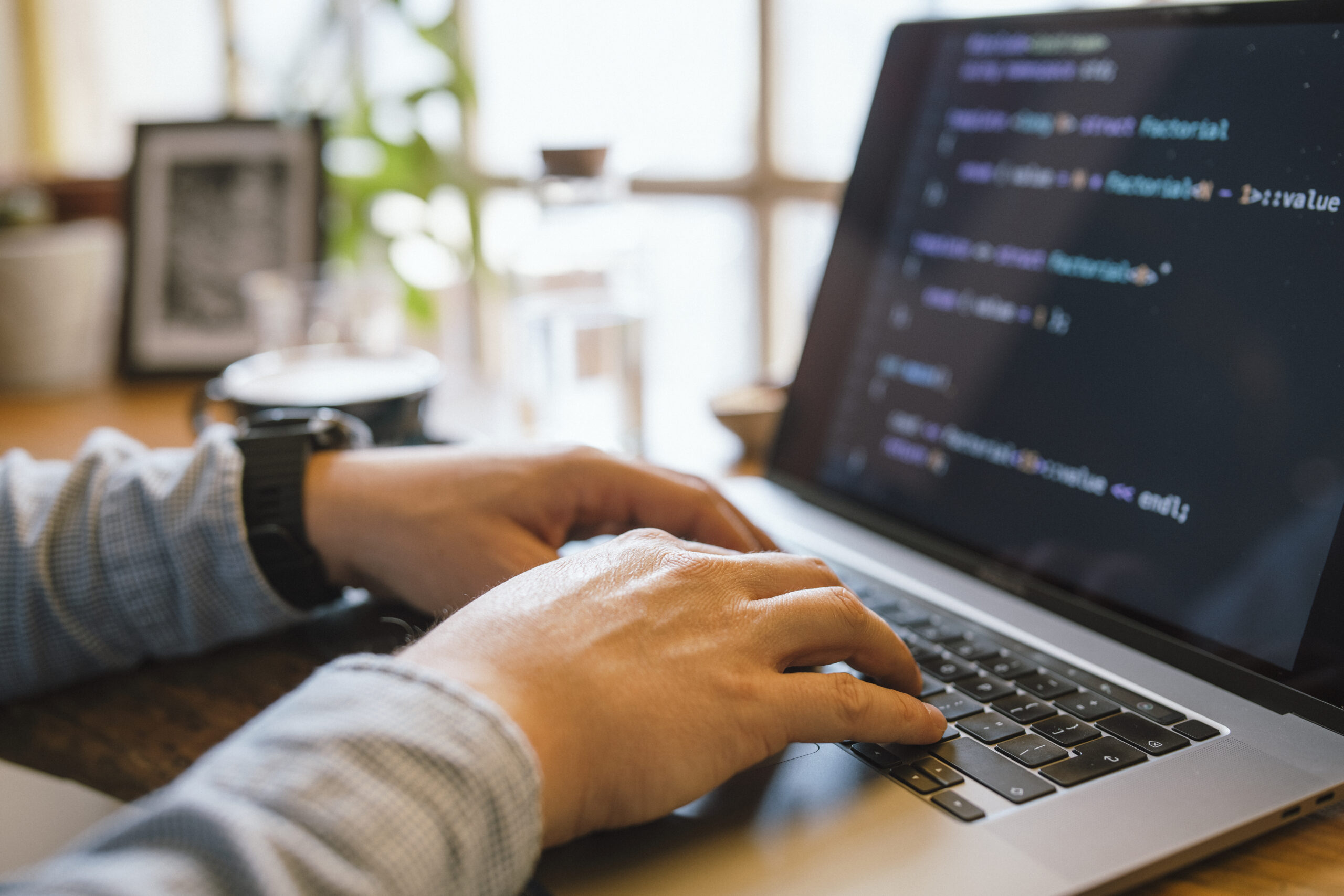
Debugging is One of the more essential — but typically forgotten — expertise in the developer’s toolkit. It isn't really pretty much fixing broken code; it’s about knowledge how and why matters go wrong, and Studying to Believe methodically to solve issues effectively. No matter whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can conserve hours of aggravation and drastically enhance your productivity. Listed here are several strategies to aid developers amount up their debugging activity by me, Gustavo Woltmann.
Learn Your Equipment
One of many quickest ways builders can elevate their debugging capabilities is by mastering the equipment they use each day. While crafting code is just one Section of improvement, understanding ways to communicate with it efficiently throughout execution is Similarly critical. Contemporary enhancement environments appear equipped with potent debugging capabilities — but lots of builders only scratch the floor of what these applications can do.
Acquire, one example is, an Integrated Growth Environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments let you set breakpoints, inspect the value of variables at runtime, step by code line by line, and also modify code on the fly. When utilised properly, they Permit you to observe accurately how your code behaves for the duration of execution, that is a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-finish builders. They enable you to inspect the DOM, keep track of community requests, view true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can switch annoying UI problems into workable responsibilities.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command more than managing procedures and memory management. Understanding these applications might have a steeper Finding out curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into comfortable with Variation Manage techniques like Git to be aware of code history, discover the exact minute bugs were being released, and isolate problematic changes.
In the end, mastering your resources signifies heading outside of default configurations and shortcuts — it’s about acquiring an personal expertise in your development environment to ensure that when concerns come up, you’re not misplaced at nighttime. The higher you recognize your equipment, the more time it is possible to devote fixing the actual issue rather than fumbling via the method.
Reproduce the challenge
The most vital — and sometimes neglected — techniques in powerful debugging is reproducing the challenge. Just before jumping in to the code or making guesses, builders will need to make a steady atmosphere or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a video game of likelihood, frequently bringing about wasted time and fragile code modifications.
The first step in reproducing a dilemma is collecting as much context as feasible. Question concerns like: What steps resulted in The problem? Which setting was it in — advancement, staging, or output? Are there any logs, screenshots, or error messages? The more depth you have, the much easier it turns into to isolate the precise problems less than which the bug happens.
As you’ve collected enough data, attempt to recreate the situation in your local natural environment. This might necessarily mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, take into account writing automated assessments that replicate the sting circumstances or point out transitions involved. These assessments don't just aid expose the situation but additionally avoid regressions in the future.
At times, The difficulty might be setting-specific — it would happen only on specified operating programs, browsers, or under certain configurations. Working with tools like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a step — it’s a attitude. It calls for tolerance, observation, in addition to a methodical approach. But after you can persistently recreate the bug, you might be already halfway to repairing it. By using a reproducible circumstance, You can utilize your debugging equipment additional effectively, test possible fixes safely and securely, and converse additional Plainly with the staff or people. It turns an summary grievance into a concrete challenge — and that’s where builders prosper.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when one thing goes Improper. Instead of seeing them as frustrating interruptions, builders really should understand to deal with error messages as immediate communications with the technique. They usually let you know precisely what happened, where by it took place, and often even why it occurred — if you know the way to interpret them.
Start out by looking through the message diligently As well as in complete. Many builders, especially when under time force, glance at the main line and promptly commence making assumptions. But further within the mistake stack or logs could lie the true root bring about. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them very first.
Break the mistake down into parts. Could it be a syntax mistake, a runtime exception, or possibly a logic mistake? Does it issue to a particular file and line selection? What module or perform induced it? These thoughts can guidebook your investigation and issue you toward the liable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Mastering to acknowledge these can dramatically hasten your debugging procedure.
Some problems are imprecise or generic, and in Individuals scenarios, it’s crucial to look at the context wherein the error transpired. Test related log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger problems and provide hints about likely bugs.
Finally, mistake messages are not your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint issues quicker, minimize debugging time, and become a a lot more successful and self-confident developer.
Use Logging Sensibly
Logging is One of the more powerful tools inside a developer’s debugging toolkit. When utilized efficiently, it provides actual-time insights into how an software behaves, supporting you fully grasp what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
A superb logging approach begins with realizing what to log and at what degree. Typical logging ranges consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic details throughout improvement, INFO for typical situations (like prosperous start off-ups), WARN for potential issues that don’t break the applying, Mistake for real problems, and Lethal if the technique can’t carry on.
Steer clear of flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure essential messages and decelerate your technique. Concentrate on key gatherings, condition changes, enter/output values, and critical conclusion factors in your code.
Structure your log messages clearly and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Specifically important in generation environments exactly where stepping by way of code isn’t possible.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, intelligent logging is about balance and clarity. By using a well-believed-out logging tactic, you are able to decrease the time it's going to take to spot difficulties, gain deeper visibility into your apps, and Increase the All round maintainability and trustworthiness of your code.
Feel Similar to a Detective
Debugging is not only a complex task—it's a kind of investigation. To proficiently identify and resolve bugs, builders will have to approach the method just like a detective resolving a mystery. This frame of mind allows stop working complicated concerns into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or performance issues. Just like a detective surveys a crime scene, collect as much relevant info as you are able to without having jumping to conclusions. Use logs, test instances, and user reports to piece together a transparent photograph of what’s occurring.
Upcoming, sort hypotheses. Question by yourself: What may be leading to this conduct? Have any adjustments a short while ago been built into the codebase? Has this problem occurred before less than very similar situation? The target is usually to slim down choices and identify probable culprits.
Then, examination your theories systematically. Make an effort to recreate the issue in a managed surroundings. If you suspect a selected operate or component, isolate it and validate if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome lead you nearer to the truth.
Fork out close notice to modest particulars. Bugs generally conceal during the minimum envisioned destinations—like a lacking semicolon, an off-by-1 mistake, or perhaps a race affliction. Be comprehensive and affected individual, resisting the urge to patch the issue devoid of totally being familiar with it. Short-term fixes may perhaps hide the true problem, just for it to resurface afterwards.
Finally, retain notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future challenges and aid Some others understand your reasoning.
By contemplating similar to a detective, builders can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering hidden concerns in advanced systems.
Compose Assessments
Producing checks is one of the best strategies to help your debugging abilities and All round growth performance. Checks don't just help catch bugs early but additionally serve as a safety net that provides you assurance when making modifications in your codebase. A effectively-examined application is simpler to debug since it permits you to pinpoint just the place and when a challenge happens.
Begin with unit exams, which concentrate on person functions or modules. These little, isolated tests can quickly expose whether a selected bit of logic is Performing as predicted. Each time a check fails, you instantly know exactly where to look, significantly lowering the time spent debugging. Device assessments are Specifically helpful for catching regression bugs—issues that reappear after Beforehand currently being set.
Subsequent, combine integration assessments and finish-to-end checks into your workflow. These support make certain that numerous aspects of your software function alongside one another efficiently. They’re specifically useful for catching bugs that come about in complex units with many elements or services interacting. If a thing breaks, your exams can show you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Consider critically regarding your code. To test a feature appropriately, you need to be aware of its inputs, expected outputs, and edge scenarios. This degree of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continually, you are able to target correcting the bug and view your take a look at move when The difficulty is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing tests turns debugging from a aggravating guessing video game right into a structured and predictable procedure—supporting you capture extra bugs, faster and even more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the trouble—observing your display screen for several hours, seeking solution following Remedy. But Among the most underrated debugging instruments is solely stepping absent. Having breaks allows you reset your thoughts, here minimize stress, and sometimes see The problem from the new point of view.
When you are way too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code which you wrote just hrs earlier. Within this state, your Mind results in being a lot less successful at dilemma-fixing. A short walk, a espresso split, and even switching to a special job for 10–quarter-hour can refresh your emphasis. Several developers report finding the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also help reduce burnout, In particular for the duration of for a longer time debugging classes. Sitting down in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping away enables you to return with renewed Electrical power in addition to a clearer frame of mind. You may instantly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, an excellent general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily beneath limited deadlines, nevertheless it basically causes a lot quicker and more effective debugging In the long term.
In a nutshell, having breaks just isn't an indication of weak spot—it’s a smart approach. It gives your brain Place to breathe, increases your viewpoint, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of resolving it.
Learn From Each and every Bug
Each individual bug you encounter is much more than simply A short lived setback—it's a chance to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a little something valuable should you make the effort to replicate and analyze what went Incorrect.
Commence by asking by yourself some vital questions once the bug is resolved: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code opinions, or logging? The responses generally expose blind places as part of your workflow or knowledge and assist you Establish much better coding patterns going ahead.
Documenting bugs can even be an outstanding practice. Retain a developer journal or retain a log where you Notice down bugs you’ve encountered, how you solved them, and what you learned. Over time, you’ll begin to see designs—recurring troubles or frequent errors—that you could proactively avoid.
In workforce environments, sharing Anything you've uncovered from a bug together with your friends is often Specially effective. Whether or not it’s via a Slack concept, a short write-up, or A fast information-sharing session, helping Other individuals stay away from the same concern boosts group performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as important portions of your advancement journey. After all, many of the ideal developers will not be the ones who publish perfect code, but people that constantly study from their errors.
In the long run, each bug you deal with adds a fresh layer towards your skill established. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging techniques takes time, follow, and endurance — but the payoff is big. It would make you a far more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become far better at That which you do.